Back-end Development with JAVA
Build Scalable, Secure, and High-Performance Applications
About the Course
If you are looking to truly master backend development and apply it in real-world projects, this course is for you. Backend Development with Java is your step-by-step guide to becoming a confident backend developer. Whether you are building RESTful APIs, working with databases, or creating scalable web applications – this course has you covered.
We will start with the basics of Java and quickly move to advanced topics like Spring Boot, Hibernate, and microservices architecture. Along the way, you will learn to build secure, efficient, and production-ready backend systems. You will also work with modern tools like Postman, and Git to streamline your development workflow.
This course includes three real-world projects: a Student Notes Application, an E-commerce Backend, and a Microservices-based Banking System. By the end of the course, you will not only be comfortable using Java for backend development but also have the confidence to apply it to real-world challenges – whether it’s building APIs, or integrating with databases.
This course is designed for anyone ready to get serious about Java backend development – whether you are just starting out or looking to expand your skills. Let’s take your backend development skills to the next level.
- Monday, 17 March 2025
- Duration: 6months
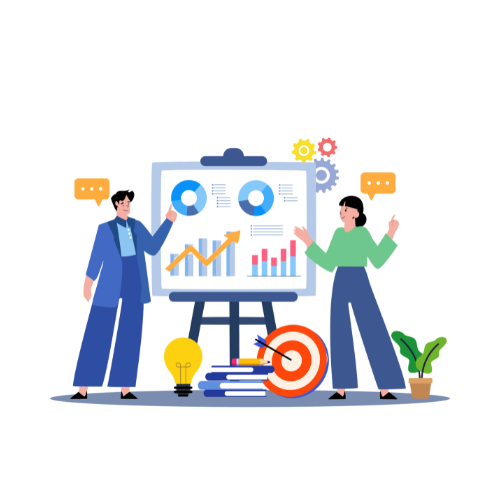
Why Enroll?
100% placement assistance
Certificate upon course completion
Resume building
Interview preparation
Experienced faculty
Why Backend Development?
● Good Job Opportunities – Backend developers are in high demand
● Lucrative Careers – Companies offer high salaries
● Foundation of Every Application – Powers modern web and mobile apps
● Scalability & Performance – Essential for building robust apps that grow and serve millions
● Versatile – Works in web, mobile, cloud, and enterprise software development
Why Java?
● Trusted by Big Companies – Popular in banks, IT firms, and enterprise applications
● Scalability & Performance – Ideal for high-traffic and mission-critical applications
● Strong Community & Ecosystem – Extensive libraries, frameworks (Spring Boot, Hibernate), and global support
● Cross-Platform Compatibility – “Write Once, Run Anywhere” makes it highly portable
About the Instructor
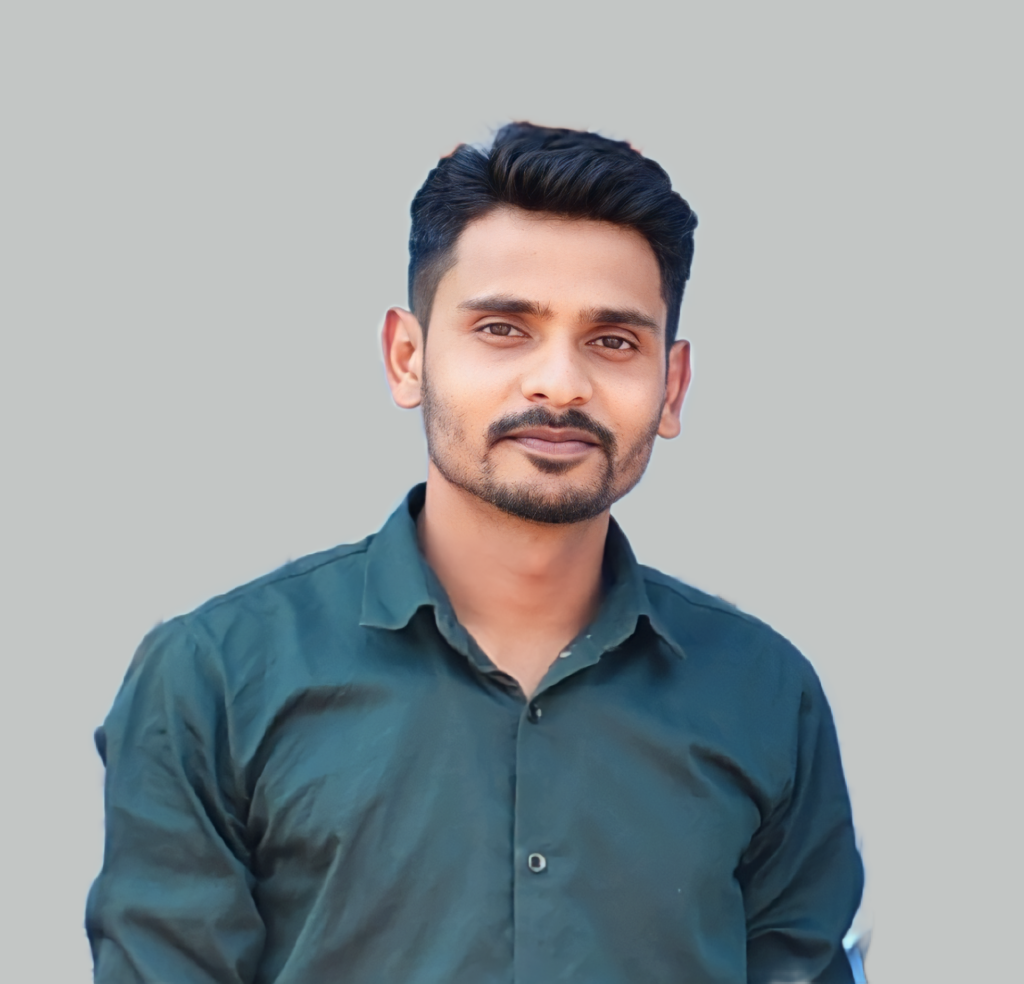
Ratnadip Tembhurkar
- M.Tech in Computer Science
- 9+ years of experience working as a Software Trainer
- ● Trained 1000+ students in software development
- ● Corporate training
- ● Trained faculties under Faculty Development Program (FDP) in multiple universities
What You Will Learn
● Write clean, efficient, and maintainable Java code.
● Build RESTful APIs using Spring Boot.
● Work with relational databases using Hibernate and JPA.
● Implement authentication and authorization using Spring Security.
● Apply microservices architecture to build scalable systems.
● Use Postman for API testing and debugging.
● Process and manage data in CSV, JSON, and XML formats.
● Implement logging and error handling in your applications.
● Use Git for version control and collaboration
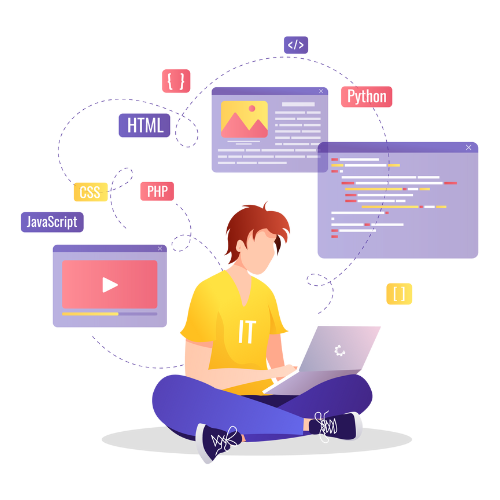
What You Will Build / Projects
1. Student Notes Application
In this project, you will build a RESTful API for a student notes application. Students can store, retrieve, update, and delete their notes. You will use Spring Boot, MySQL Database, and Spring Data JPA to create a fully functional backend system.
2. E-commerce Backend
Together, we will develop the backend for an e-commerce platform. You will implement features like product management, order processing, and user authentication. This project will teach you to work with MySQL, Spring Security, and RESTful APIs.
3. Microservices-based Banking System
In this advanced project, you will build a banking system using microservices architecture. You will create separate services for user management, transactions, and notifications.
Course Content
Core Java (60h)
1. Java – Why? What? How? When? Where?
2. How Java is different from other technologies
1. How to Install & set PATH
2. A Simple Java Program
3. Compiling & executing Java Program
4. Phases of Java Program
5. Analysis of a Java Program
6. Understanding Syntax and Semantic Error
7. Runtime Exception
8. Name of a Java Source File
9. Platform Independency
10. Java Technology (JDK, JRE, JVM, JIT)
11. Features of Java
12. Text Editors
13. Consoles
1. Naming conventions in Java
2. Comments
3. Statements
4. Blocks (Static, Non-static / instance)
5. Identifiers
6. Keywords
7. Literals
8. Primitive Data Types, Range
9. Reference (User defined) Data type
10. Variables (Primitive, Reference)
11. Type Casting, Default Value
12. Operators
13. Interview related Questions and Answers
- 1. Working with Control Structures
2. Types of Control Structures
3. Decision Control Structure (if, if-else, if else if, switch case)
4. Repetition Control Structure (do while,while, for)
5. Interview related Questions and Answers
1. Java program inputs from Keyboard
2. Methods of Keyboard inputs
3. Scanner, Buffered Reader
4. Problem Solving
5. Java Array
6. What is Array
7. Array Declaration in java vs C and C++.
8. Instantiation of an Array
9. Multi-dimensional Arrays
10. Interview related Questions and Answers
1. Procedural vs Object Oriented Program
2. Introduction to Object Oriented
3. Abstraction, Encapsulation, Inheritance, Polymorphism
4. Introduction to Classes and Objects
5. Instance and Static Variables
6. Different ways to create Object Instance
7. Instance Variable and its role in a Class
8. Constructors
9. Constructors, types of Constructor, Constructor Rule, Constructor
Overloading
10. Static Variable and its use
11. Methods and their behavior
12. Constructor vs Methods
13. Java Access Modifiers (and Specifiers)
14. Interview related Questions and Answers
1. Using various Editors
2. Program Compilation, Execution in Editor
3. Using Eclipse IDE
4. Project Set Up
5. Source File Generation
6. Application Compilation and Run
1. Complete concepts of Inheritance
2. Sub-Classes
3. Object Classes
4. Constructor Calling Chain
5. The use of “super” Keyword
6. The use of “private” keyword inheritance.
7. Reference Casting
1. Introduction to Abstract Methods
2. Abstract Classes and Interface
3. Interface as a Type
4. Interface vs Abstract Class
5. Interface Definition
6. Interface Implementation
7. Multiple Interfaces Implementation
8. Interfaces Inheritance
9. How to create object of Interface
1. Introduction to Polymorphism
2. Types of Polymorphism
3. Overloading Methods
4. Overriding Methods
5. Hiding Methods
6. Final Class and Method
7. Interview related Questions and Answers
- 1. Package and Class path and its use
2. Package Creation and Use
3. Class Import
4. Package Import
5. Role of public, protected, default and private w.r.t package
1. Java.lang Hierarchy
2. Object class and using toString(), equals(), hashCode(), clone(),
finalize(), etc.
3. Using Runtime Class, Process Class to play music, video from Java
Program
4. Primitives and Wrapper Class
5. Math Class
6. String, StringBuffer, StringBuilder Class
7. String Constant Pool
8. Various usage and methods of String, StringBuffer, StringBuilder
9. Wrapper Classes
10. System Class using gc(), exit(), etc
1. Auto boxing and Auto unboxing
2. Static import
3. Instance of operator
4. Enum and its use in Java
5. Working with jar
1. Garbage Collection Introduction
2. Advantages of Garbage Collection
3. Garbage Collection Procedure
4. Java API
5. Interview related Questions and Answers
1. Introduction to Exceptions
2. Effects of Exceptions
3. Exception Handling Mechanism
4. Try, catch, finally blocks
5. Rules of Exception Handling
6. Exception class Hierarchy, Checked & Unchecked Exception
7. Throw & throws keyword
8. Custom Exception Class
9. Interview related Questions and Answers
1. Introduction
2. Advantages
3. Creating a Thread by inheriting from Thread class
4. Run() and start() method
5. Constructor of Thread Class
6. Various Method of Thread Class
7. Runnable Interface Implementation
8. Life Cycle of Thread
9. Interview related Questions and Answers
1. Java I/O Stream
2. I/O Stream – Introduction
3. Types of Streams
4. Stream Class Hierarchy
5. Using File Class
6. Copy and Paste the content of a file
7. Byte Streams vs Character Streams
8. Text File vs Binary File
9. Character Reading from Keyboard by Input Stream Reader
10. Reading a Line/String from Keyboard by Buffered Reader
11. Standard I/O Streams Using Data Streams to read/write
12. Interview related Questions and Answers
1. What is Collection Framework
2. List, Set & Map interfaces
3. Using Vector, Array List, Stack, Linked List, etc.
4. Using Collections class for sorting
5. Using Hashtable, Hash Map, Tree Map, SortedMap, LinkedHashMap
etc.
6. Iterator, Enumerator.
7. Using Queue, Deque, SortedQue, etc.
8. Using HashSet, TreeSet, LinkedHashSet etc.
9. Interview related Questions and Answers
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Optio, neque qui velit. Magni dolorum quidem ipsam eligendi, totam, facilis laudantium cum accusamus ullam voluptatibus commodi numquam, error, est. Ea, consequatur.
Advanced Java (54h)
1. Introduction to JDBC
2. Databases and Drivers
3. Types of Driver
4. Loading a driver class file
5. Establishing Connection to different Database with different Driver
6. Executing SQL queries by ResultSet, Statements, PreparedStatment
interface
7. Using CallableStatement
8. Transaction Management & BatchUpdate
9. Interview related Questions and Answers
1. Introduction to Servlet
2. Servlet life cycle and Request processing
3. Handling forms and user inputs
4. Session management
5. Integration with database
6. Interview related Questions and Answers
1. Introduction to JSP and its advantages
2. JSP architecture and page directives
3. Scripting elements
4. Standard action tags
5. JSTL
6. Interview related Questions and Answers
1. Developing RESTful web services
2. JSON
1. Introduction to ORM
2. Hibernate framework overview
3. Mapping entities to database tables
4. Operations using hibernate
5. Inbuilt functions of hibernate
6. Criteria and query
7. HQL
8. Solving company use cases
9. Interview related Questions and Answers
1. Introduction to Spring
2. Dependency Injection and Inversion of Control (IoC)
3. Spring annotation
4. Database integration with Spring
5. Aspect-Oriented programming with Spring
6. Interview related Questions and Answers
1. Creating Spring boot application
2. Dependency Injection
3. Spring boot annotation
4. Auto-configuration and started dependencies
5. HTTP methods
6. Spring boot hibernate integration
7. Building RESTful APIs with Spring boot
8. Solving Company use cases
1. Introduction to microservices architecture
2. Controller and REST endpoints
3. Returning Plain Text or JSON response
4. API testing using POSTMAN
5. application.properties and configuration
6. Hands on practice with projects
7. Interview related Questions and Answers
1. Understanding MVC Architecture
2. Implementing MVC with Spring boot
3. Developing web application
4. Separation of Layers
5. Interview related Questions and Answers
1. Introduction
2. Database Fundamental
3. SQL Essentials
4. Installation of MySQL
5. Exploring SQL Statements
6. Interview related Questions and Answers
7. Advance Java Tools & Technology
8. Apache Maven
9. Tomcat Server
10. Unit Testing
11. Postman